In today’s digital landscape, securing your web applications is more critical than ever. One of the most common security threats is Cross-Site Scripting (XSS) attacks. XSS vulnerabilities can compromise the integrity of your web applications and put your users at risk. This blog post will explore how to secure your JavaScript code and protect your web applications from XSS attacks.
1. Understanding Cross-Site Scripting (XSS)
Cross-Site Scripting (XSS) is a vulnerability that allows attackers to inject malicious scripts into webpages viewed by other users. These scripts can steal sensitive information, manipulate the DOM, or perform actions on behalf of the user. There are three main types of XSS attacks:
- Stored XSS: Malicious scripts are permanently stored on the server (e.g., in a database) and executed when users access the affected page.
- Reflected XSS: Malicious scripts are included in a URL or request and executed immediately when the user clicks on the link or submits the form.
- DOM-Based XSS: Malicious scripts are executed as a result of client-side JavaScript manipulating the DOM, without any server-side involvement.
2. Best Practices for Securing JavaScript Code
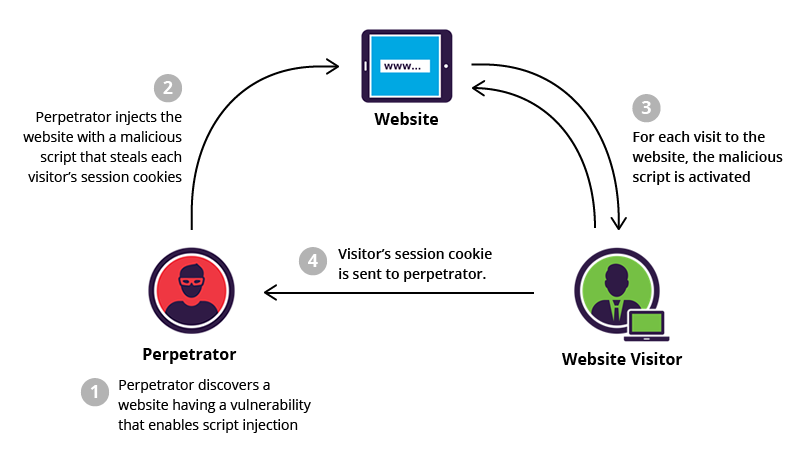
Securing your JavaScript code involves implementing best practices to mitigate XSS and other vulnerabilities. Here are some essential strategies:
1. Input Validation and Sanitization:
- Validate User Input: Ensure that all user inputs are validated both on the client side and server side. Use data types and length constraints to prevent invalid data from being processed.
- Sanitize Input Data: Sanitize user inputs to remove any potentially harmful characters or scripts. Use libraries like DOMPurify to clean HTML and prevent XSS attacks.
2. Use Content Security Policy (CSP):
- Implement CSP: Content Security Policy (CSP) is a security feature that helps prevent XSS attacks by restricting the sources from which content can be loaded. Configure CSP headers to allow only trusted sources and block inline scripts.
3. Escape User Output:
- Escape Output Data: When displaying user-generated content, escape special characters to prevent them from being interpreted as HTML or JavaScript. Use libraries or built-in functions to encode characters like
<
,>
, and&
.
4. Avoid Inline JavaScript:
- Externalize JavaScript Code: Avoid placing JavaScript code directly in HTML files. Instead, use external scripts and link them through script tags. This approach helps CSP policies and reduces the risk of inline script execution.
5. Implement Secure Cookie Practices:
- Set Secure and HttpOnly Flags: Ensure that cookies used for authentication or sensitive information are marked as
Secure
andHttpOnly
. TheSecure
flag ensures cookies are only sent over HTTPS, while theHttpOnly
flag prevents JavaScript from accessing cookie data.
6. Regularly Update Dependencies:
- Keep Libraries Updated: Regularly update third-party libraries and dependencies to their latest versions. Security patches and updates often address vulnerabilities that could be exploited by attackers.
3. Example: Mitigating XSS in a Simple Web Application
Let’s consider a simple web application that allows users to submit comments. Without proper security measures, this application could be vulnerable to XSS attacks.
Vulnerable Code Example:
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Comment Section</title>
</head>
<body>
<form id="commentForm">
<textarea id="comment" placeholder="Enter your comment"></textarea>
<button type="submit">Submit</button>
</form>
<div id="comments"></div>
<script>
document.getElementById('commentForm').addEventListener('submit', function(event) {
event.preventDefault();
const comment = document.getElementById('comment').value;
document.getElementById('comments').innerHTML += `<p>${comment}</p>`;
});
</script>
</body>
</html>
In the above example, user input is directly inserted into the DOM, which could allow an attacker to inject malicious scripts.
Secured Code Example:
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Comment Section</title>
</head>
<body>
<form id="commentForm">
<textarea id="comment" placeholder="Enter your comment"></textarea>
<button type="submit">Submit</button>
</form>
<div id="comments"></div>
<script>
function escapeHtml(unsafe) {
return unsafe
.replace(/&/g, "&")
.replace(/</g, "<")
.replace(/>/g, ">")
.replace(/"/g, """)
.replace(/'/g, "'");
}
document.getElementById('commentForm').addEventListener('submit', function(event) {
event.preventDefault();
const comment = document.getElementById('comment').value;
const safeComment = escapeHtml(comment);
document.getElementById('comments').innerHTML += `<p>${safeComment}</p>`;
});
</script>
</body>
</html>
In this secured version, the escapeHtml
function is used to sanitize user input before displaying it, preventing potential XSS attacks.
4. Conclusion
Securing your JavaScript code and protecting against XSS attacks is crucial for maintaining the integrity and safety of your web applications. By implementing best practices such as input validation, escaping output, using Content Security Policy (CSP), and avoiding inline JavaScript, you can significantly reduce the risk of XSS vulnerabilities. Always stay vigilant and keep your codebase and dependencies up to date to ensure a secure user experience.
By taking these precautions, you not only protect your users from potential security threats but also enhance the overall reliability and trustworthiness of your web applications.