Custom overlays in JavaScript can significantly enhance web maps by providing additional layers of information and interactivity. These overlays can include custom markers, polygons, pop-ups, heat maps, and more, allowing developers to create rich, informative, and engaging map experiences.
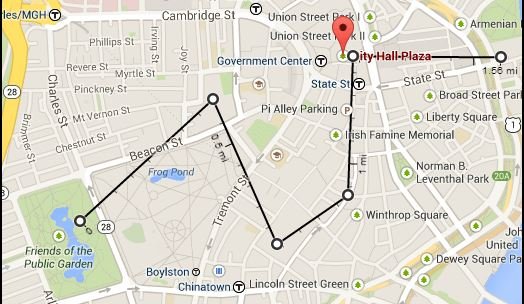
Understanding Custom Overlays
Custom overlays are additional layers added to a base map to display extra information. These can be anything from simple markers to complex data visualizations. They are essential for providing context-specific data and improving the user experience by making maps more interactive and informative.
Popular Libraries for Custom Overlays
Several JavaScript libraries offer robust features for adding custom overlays to maps. The most popular ones include Leaflet, Mapbox GL JS, and the Google Maps API.
Leaflet: Leaflet is a lightweight library that is highly flexible and easy to use. It supports a variety of overlays, including markers, circles, polygons, and custom layers. Leaflet’s extensive plugin ecosystem allows developers to add specialized overlays, such as heat maps and clustering.
Mapbox GL JS: Mapbox GL JS provides high-performance, vector-tiled maps and supports complex custom overlays. It allows for data-driven styling and interactive data visualization. Custom overlays in Mapbox GL JS can be created using GeoJSON data, making it easy to integrate dynamic and rich overlays.
Google Maps API: The Google Maps API is a comprehensive tool for adding custom overlays. It supports markers, polylines, polygons, custom tiles, and more. The API’s versatility and extensive documentation make it suitable for complex custom overlay implementations.
Implementing Custom Overlays
To implement custom overlays, follow these steps:
- Choose a Library: Select a JavaScript library that suits your project’s needs. Consider factors such as ease of use, performance, and available features.
- Initialize the Map: Set up your map using the chosen library. Configure basic settings like the map center, zoom level, and base layers.
- Create Overlay Data: Define the data for your overlays. This could be GeoJSON for geographic data, arrays of coordinates for markers, or any other relevant data structure.
- Add Overlays: Use the library’s API to add your overlays. This could involve creating marker instances, drawing polygons, or adding custom HTML elements as overlays.
- Customize Appearance and Behavior: Style your overlays and add interactivity. Use CSS for styling and JavaScript for adding event listeners and other behaviors.
- Optimize and Test: Ensure that your overlays perform well and provide a smooth user experience. Test on various devices and browsers to ensure compatibility.
Example: Adding Custom Overlays with Leaflet
Here’s a basic example of adding custom overlays using Leaflet:
<html>
<head>
<title>Leaflet Custom Overlays</title>
<link rel="stylesheet" href="https://unpkg.com/leaflet/dist/leaflet.css" />
<style>
#map { height: 500px; }
</style>
</head>
<body>
<div id="map"></div>
<script src="https://unpkg.com/leaflet/dist/leaflet.js"></script>
<script>
// Initialize the map
var map = L.map('map').setView([51.505, -0.09], 13);
// Add a base layer
L.tileLayer('https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png', {
maxZoom: 19
}).addTo(map);
// Define custom overlay data
var customMarker = L.marker([51.5, -0.09]).bindPopup('A Custom Marker');
var customCircle = L.circle([51.508, -0.11], {
color: 'red',
fillColor: '#f03',
fillOpacity: 0.5,
radius: 500
}).bindPopup('A Custom Circle');
// Add overlays to the map
customMarker.addTo(map);
customCircle.addTo(map);
</script>
</body>
</html>
Best Practices for Custom Overlays
When creating custom overlays, consider the following best practices:
- Optimize Performance: Ensure overlays do not negatively impact map performance. Use clustering for large datasets and optimize rendering.
- Maintain Usability: Ensure that overlays do not clutter the map and that important map features remain visible and accessible.
- Ensure Responsiveness: Test overlays on various devices and screen sizes to ensure a consistent user experience.
- Provide Interactivity: Use pop-ups, tooltips, and other interactive elements to make overlays informative and engaging.
Conclusion
Custom overlays in JavaScript enhance web maps by adding layers of rich, interactive information. By leveraging libraries like Leaflet, Mapbox GL JS, and Google Maps API, developers can create dynamic and engaging map experiences tailored to their users’ needs.