In the dynamic world of JavaScript development, typing plays a crucial role in writing reliable and maintainable code. While JavaScript is a dynamically typed language, TypeScript introduces a static typing system that offers numerous advantages. In this blog post, we will explore the basics of TypeScript and JavaScript typing, and how TypeScript can enhance your development experience.
1. Understanding JavaScript Typing
JavaScript is known for its flexibility with data types. It is dynamically typed, meaning that variables can hold any type of value (string, number, object, etc.), and their type can change at runtime. This flexibility is powerful but can lead to unexpected bugs and challenges, especially in large codebases.
Example of Dynamic Typing:
let variable = "Hello, World!";
console.log(typeof variable); // string
variable = 42;
console.log(typeof variable); // number
Explanation:
- In JavaScript, the type of
variable
can change from a string to a number without errors. - This flexibility can cause issues when functions or operations expect specific types.
2. Introduction to TypeScript
TypeScript is a statically typed superset of JavaScript developed by Microsoft. It adds type annotations to JavaScript, allowing developers to specify the types of variables, function parameters, and return values. TypeScript is designed to help catch errors early in the development process and improve code quality and maintainability.
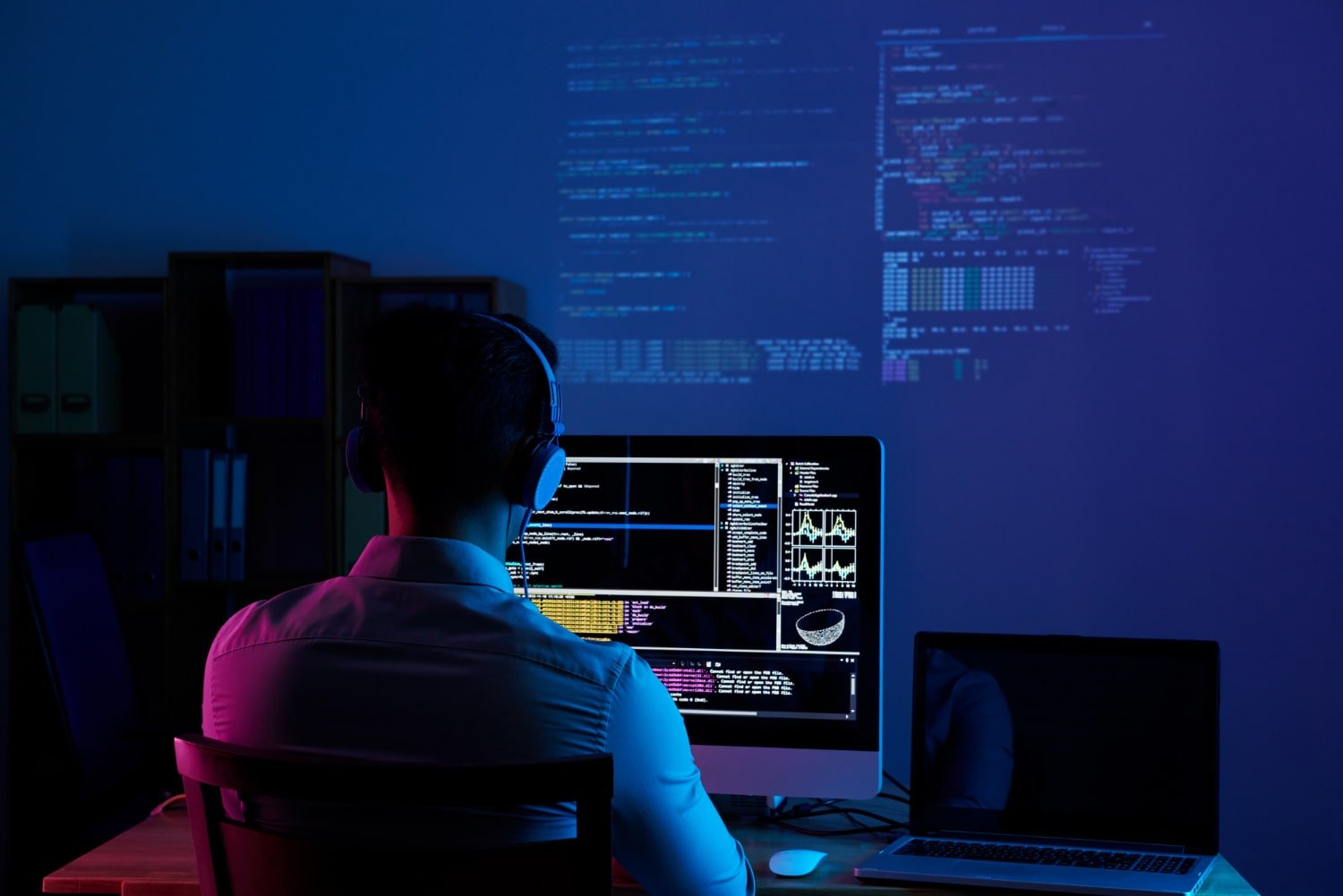
Key Features of TypeScript:
- Static Typing: TypeScript allows you to define types for variables, function parameters, and return values, providing early error detection.
- Type Inference: TypeScript can infer types based on the code, reducing the need for explicit type annotations.
- Enhanced IDE Support: TypeScript offers better code completion and refactoring tools in integrated development environments (IDEs).
3. Basic TypeScript Syntax
TypeScript extends JavaScript with additional syntax for type annotations. Here’s a simple example to illustrate how TypeScript enhances JavaScript with typing:
TypeScript Example:
function greet(name: string): string {
return `Hello, ${name}!`;
}
const message: string = greet("Alice");
console.log(message); // Hello, Alice!
Explanation:
- The
greet
function is annotated withname: string
and: string
for the return type, specifying that the function takes a string parameter and returns a string. - The
message
variable is explicitly typed as astring
.
4. Benefits of Using TypeScript
- Early Error Detection: TypeScript’s static type checking helps identify type-related errors during development, reducing runtime errors.
- Improved Code Readability: Type annotations make the code more readable and self-documenting, helping developers understand the expected types of values and functions.
- Better Tooling: TypeScript provides advanced tooling features, such as autocompletion and refactoring, which enhance the development experience.
5. TypeScript vs. JavaScript
While JavaScript remains the core language for web development, TypeScript offers an enhanced development experience through its static typing system. Here’s a quick comparison:
- JavaScript: Dynamically typed, flexible, no type annotations, potential for runtime errors.
- TypeScript: Statically typed, type annotations, compile-time error checking, improved code quality.
6. Getting Started with TypeScript
To start using TypeScript, you need to install it and set up your development environment:
- Install TypeScript: Use npm to install TypeScript globally or locally in your project.
bash
npm install -g typescript
- Compile TypeScript: Use the TypeScript compiler (
tsc
) to compile.ts
files into JavaScript.bashtsc yourfile.ts
- Configure TypeScript: Create a
tsconfig.json
file to configure TypeScript options for your project.
7. Conclusion
TypeScript enhances JavaScript development by introducing static typing, which helps catch errors early and improve code quality. By leveraging TypeScript’s features, developers can write more robust and maintainable code. Whether you are starting a new project or integrating TypeScript into an existing codebase, its benefits can significantly enhance your development workflow.
Embracing TypeScript is a step towards better software engineering practices and can lead to more reliable and scalable applications.