JavaScript is one of the most versatile and widely-used programming languages in web development. It empowers developers to create interactive, dynamic, and high-performance web applications. Whether you’re a beginner or an experienced coder, mastering JavaScript can significantly enhance your programming skills. This guide will take you through the essentials of JavaScript, from basic syntax and structures to more advanced techniques.
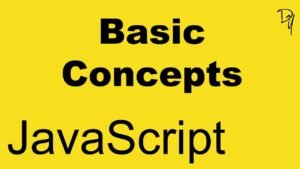
1. Understanding JavaScript Fundamentals
Before diving into complex topics, it’s crucial to grasp the fundamentals of JavaScript. This section covers:
- Variables and Data Types: Learn about JavaScript’s primitive data types (string, number, boolean, null, undefined, symbol) and how to declare variables using
var
,let
, andconst
. - Operators: Explore arithmetic, comparison, logical, and assignment operators, and understand how they work.
- Control Structures: Master conditional statements (
if
,else
,switch
) and loops (for
,while
,do...while
) to control the flow of your code.
2. Functions and Scope
Functions are a core aspect of JavaScript programming. This section will teach you:
- Function Declaration and Expressions: Understand the differences between function declarations and expressions, and learn about arrow functions.
- Scope and Closures: Discover the concepts of function scope, block scope, and how closures work in JavaScript.
3. Object-Oriented Programming in JavaScript
JavaScript supports object-oriented programming (OOP) principles, which are essential for creating modular and reusable code. This section includes:
- Objects and Prototypes: Learn about creating objects using literals and constructors, and understand prototype inheritance.
- Classes and Inheritance: Explore ES6 classes, how to define them, and how inheritance works with classes.
4. Asynchronous JavaScript
Handling asynchronous operations is crucial in modern web development. This section covers:
- Callbacks: Understand how to use callbacks to handle asynchronous tasks.
- Promises: Learn about Promises and how they help manage asynchronous operations more effectively.
- Async/Await: Discover how
async
andawait
simplify working with asynchronous code.
5. JavaScript in the Browser
JavaScript’s interaction with the browser is fundamental for web development. This section includes:
- DOM Manipulation: Learn how to use JavaScript to manipulate the Document Object Model (DOM) to create dynamic web pages.
- Event Handling: Explore how to handle user events such as clicks, form submissions, and keyboard inputs.
- AJAX and Fetch API: Understand how to make asynchronous HTTP requests to interact with servers and load data dynamically.
6. Advanced JavaScript Techniques
For those looking to deepen their JavaScript knowledge, this section covers:
- ES6+ Features: Discover new features introduced in ES6 and later versions, such as destructuring, template literals, and modules.
- Design Patterns: Learn about common design patterns used in JavaScript, including Singleton, Module, and Observer patterns.
- Performance Optimization: Explore techniques to optimize JavaScript performance and improve the efficiency of your code.
Conclusion
Mastering JavaScript requires practice and a solid understanding of its core concepts and advanced techniques. By following this guide, you’ll build a strong foundation and gain the skills needed to tackle complex web development projects. Continue to experiment with new features, tools, and best practices to stay ahead in the ever-evolving world of JavaScript.
Additional Resources
For further learning, consider exploring online tutorials, documentation, and community forums. Engaging with the JavaScript community can provide valuable insights and support as you continue your journey.