Unit testing is a fundamental practice in software development that ensures the reliability and correctness of individual units of code. In the context of JavaScript, unit testing involves writing tests for functions, methods, or components to verify their behavior under various conditions. By implementing unit tests, developers can identify and fix bugs early in the development process, leading to more robust and maintainable code.
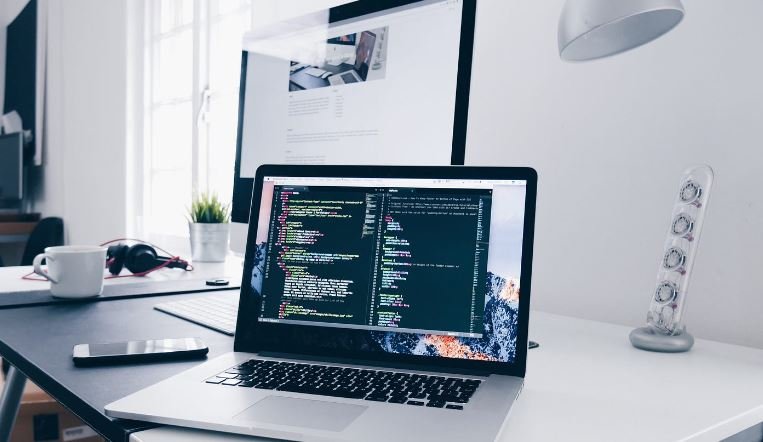
Benefits of Unit Testing
Unit testing offers numerous benefits that contribute to the overall quality of a JavaScript application. First and foremost, it provides a safety net that catches regressions and unintended changes. When new features are added or existing code is modified, unit tests help ensure that the changes do not break the application. Additionally, unit tests serve as documentation, clearly outlining the expected behavior of the code. This makes it easier for new developers to understand the codebase and for teams to maintain consistency in their coding standards.
Popular JavaScript Testing Frameworks
Several testing frameworks are available to facilitate unit testing in JavaScript. Jest, developed by Facebook, is a widely-used framework known for its simplicity and powerful features. It offers a comprehensive set of tools for writing, running, and debugging tests, making it a popular choice for React applications. Mocha, another popular framework, provides a flexible and customizable testing environment. It works well with other libraries like Chai for assertions and Sinon for mocks and spies. Jasmine is also a well-known framework, particularly favored for its behavior-driven development (BDD) approach and its ease of use.
Writing Effective Unit Tests
Writing effective unit tests involves several key principles. Tests should be isolated, meaning each test should focus on a single unit of code and should not depend on external factors like the network or database. This isolation ensures that tests run quickly and consistently. Additionally, tests should be deterministic, producing the same results every time they are run. This is achieved by controlling the inputs and mocking external dependencies. Clear and descriptive test names are essential, as they provide insight into the purpose and expected outcome of the tests. Lastly, strive for comprehensive test coverage by writing tests that cover various scenarios, including edge cases and error conditions.
Test-Driven Development (TDD)
Test-Driven Development (TDD) is a development methodology that emphasizes writing tests before writing the actual code. In TDD, developers start by writing a test for a new feature or functionality, ensuring that the test initially fails. Then, they write the minimal amount of code required to pass the test. Once the test passes, they refactor the code to improve its structure while ensuring that all tests still pass. TDD promotes a disciplined approach to coding, resulting in cleaner, more reliable code and a comprehensive test suite that serves as a form of living documentation.
Continuous Integration and Testing
Integrating unit tests into a continuous integration (CI) pipeline is crucial for maintaining code quality in larger projects. CI systems automatically run unit tests whenever code changes are committed to the repository, providing immediate feedback to developers. This practice ensures that new code does not introduce regressions and that the application remains stable. Popular CI tools like Jenkins, Travis CI, and GitHub Actions support running JavaScript unit tests as part of the build process, facilitating seamless integration and deployment workflows.
Conclusion
Unit testing is an essential practice for ensuring the reliability and maintainability of JavaScript applications. By leveraging popular testing frameworks like Jest, Mocha, and Jasmine, developers can write effective unit tests that catch bugs early and provide a safety net for future code changes. Embracing Test-Driven Development (TDD) and integrating tests into a continuous integration pipeline further enhances code quality and promotes a disciplined approach to development. Mocking and stubbing techniques enable isolated testing of units, ensuring that tests run quickly and consistently. Ultimately, unit testing leads to more robust and high-quality JavaScript applications.