Design patterns are reusable solutions to common problems encountered during software development. In JavaScript, design patterns help developers write code that is modular, maintainable, and scalable. In this blog post, we’ll explore some of the most essential JavaScript design patterns and how you can use them to enhance your coding practices.
1. Introduction to Design Patterns
Design patterns provide proven solutions to recurring design issues. They help developers avoid reinventing the wheel and ensure that code adheres to best practices. JavaScript design patterns are particularly useful due to the language’s flexibility and wide range of use cases.
2. Singleton Pattern
The Singleton pattern ensures a class has only one instance and provides a global point of access to it. This pattern is useful for managing a shared resource, such as a configuration or connection pool.
Example: Singleton Pattern
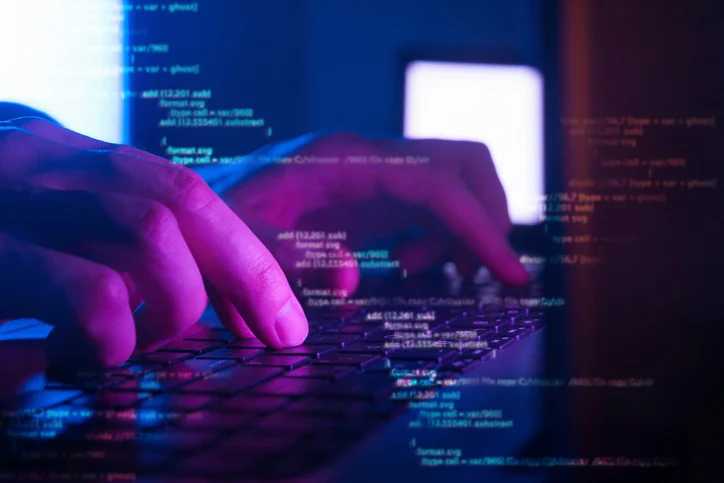
const Singleton = (function () {
let instance;
function createInstance() {
return { message: "I am the singleton instance!" };
}
return {
getInstance: function () {
if (!instance) {
instance = createInstance();
}
return instance;
}
};
})();
// Usage
const instance1 = Singleton.getInstance();
const instance2 = Singleton.getInstance();
console.log(instance1 === instance2); // true
Explanation:
- The Singleton pattern is implemented using an immediately invoked function expression (IIFE) to create a single instance.
- The
getInstance
method returns the existing instance or creates a new one if it doesn’t exist.
3. Factory Pattern
The Factory pattern defines an interface for creating objects but allows subclasses to alter the type of objects that will be created. This pattern is ideal for situations where a system needs to create objects from a family of related classes.
Example: Factory Pattern
class Car {
constructor(make) {
this.make = make;
}
}
class CarFactory {
static createCar(make) {
return new Car(make);
}
}
// Usage
const myCar = CarFactory.createCar("Toyota");
console.log(myCar.make); // Toyota
Explanation:
- The Factory pattern uses a
CarFactory
class to createCar
objects. - This pattern simplifies the creation process and encapsulates the logic for creating objects.
4. Module Pattern
The Module pattern provides a way to encapsulate private variables and methods within a closure, exposing only the public API. This pattern helps in organizing code into reusable modules and avoids polluting the global namespace.
Example: Module Pattern
const CounterModule = (function () {
let count = 0;
return {
increment: function () {
count++;
console.log(count);
},
decrement: function () {
count--;
console.log(count);
}
};
})();
// Usage
CounterModule.increment(); // 1
CounterModule.increment(); // 2
CounterModule.decrement(); // 1
Explanation:
- The Module pattern uses an IIFE to create a private scope.
- It exposes only the methods needed to interact with the private variables, ensuring encapsulation.
5. Observer Pattern
The Observer pattern defines a one-to-many dependency between objects, allowing one object to notify multiple observers about changes. This pattern is often used in event-driven programming.
Example: Observer Pattern
class Subject {
constructor() {
this.observers = [];
}
addObserver(observer) {
this.observers.push(observer);
}
notifyObservers(message) {
this.observers.forEach(observer => observer.update(message));
}
}
class Observer {
update(message) {
console.log(`Observer received: ${message}`);
}
}
// Usage
const subject = new Subject();
const observer1 = new Observer();
const observer2 = new Observer();
subject.addObserver(observer1);
subject.addObserver(observer2);
subject.notifyObservers("Hello, observers!");
Explanation:
- The Observer pattern allows the
Subject
to notify all registeredObserver
instances of changes. - This pattern is useful for implementing event systems and notifications.
6. Prototype Pattern
The Prototype pattern creates objects based on a template of an existing object through cloning. This pattern is useful for scenarios where creating new instances from scratch is costly.
Example: Prototype Pattern
const CarPrototype = {
init(make, model) {
this.make = make;
this.model = model;
},
getDetails() {
return `${this.make} ${this.model}`;
}
};
function createCar(make, model) {
const car = Object.create(CarPrototype);
car.init(make, model);
return car;
}
// Usage
const myCar = createCar("Ford", "Mustang");
console.log(myCar.getDetails()); // Ford Mustang
Explanation:
- The Prototype pattern uses
Object.create
to clone the prototype and create new instances. - This approach is efficient for creating objects with similar properties and methods.
7. Conclusion
JavaScript design patterns offer robust solutions for common development challenges, enabling developers to write clean, maintainable, and efficient code. By understanding and applying patterns such as Singleton, Factory, Module, Observer, and Prototype, you can enhance your software architecture and improve code reusability.
Whether you’re managing global state, creating objects, or handling events, leveraging these design patterns will lead to better structured and more scalable applications.
4o mini